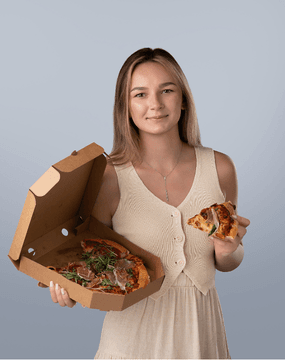
By Anita, May 22, 2025 · 7 min read
10 Alpine.js Tricks Every Magento Developer Should Know (Hyvä Edition)
Introduction
Hey there, Magento developer!
Alpine.js is a small but powerful JavaScript framework - perfect for Magento stores using Hyvä themes. It helps you build interactive features without writing a lot of JavaScript. In this post, I’ll share 10 practical tricks based on my experience working with Hyvä and real Magento projects. These are things that helped us improve performance, simplify code, and create better user experiences.
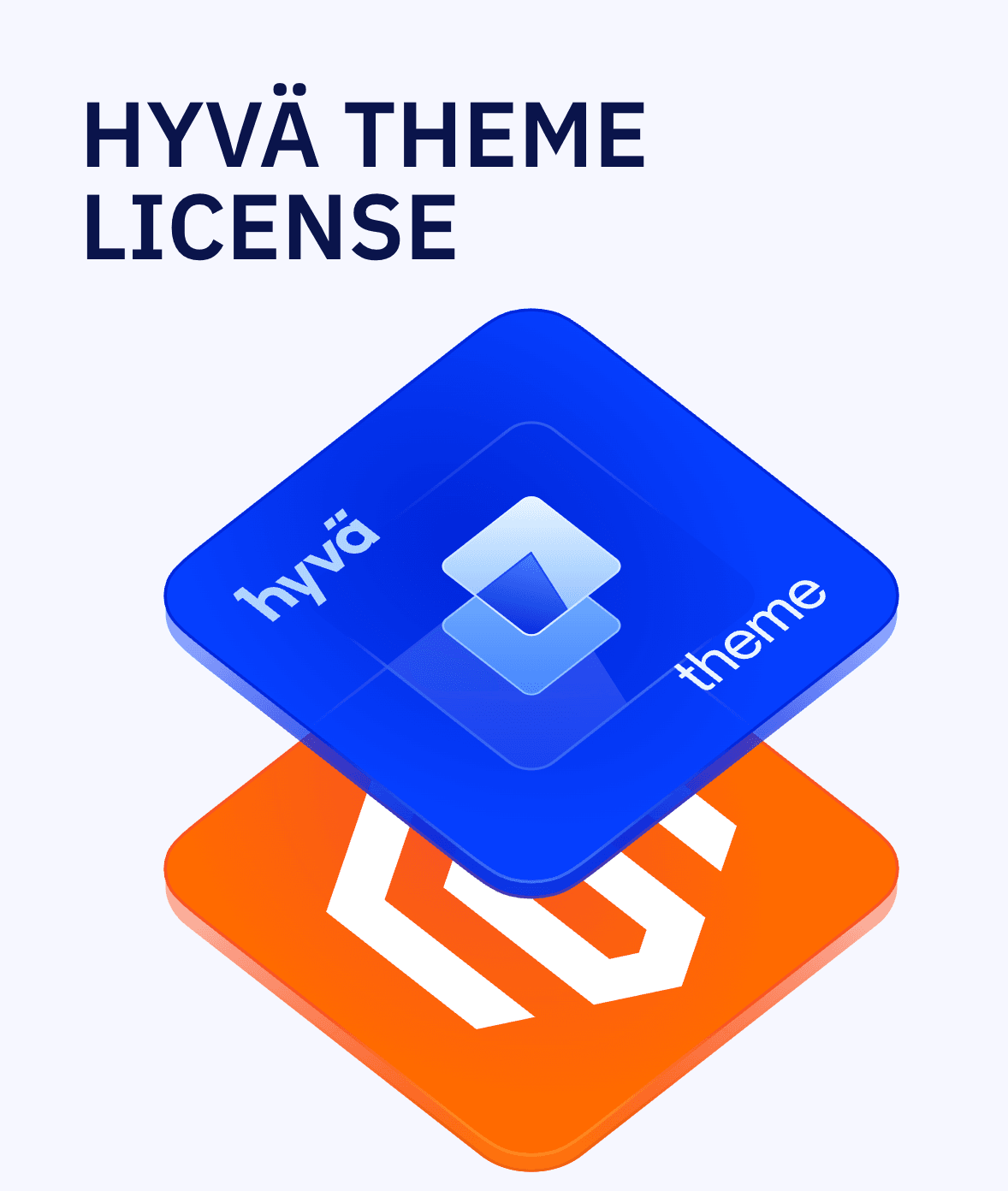
10 Useful Alpine.js Tricks
If you’re using the Hyvä theme, you already know Alpine.js keeps your front end feeling fresh without loading a big framework. Did you know that Hyva and Alpine have some handy tricks that can make your work even easier? Whether you’re building new features or fine-tuning existing ones, these tips will help you write cleaner code and move faster.
1. Hyvä Modals – Easy Pop-Ups
Hyva gives you a super simple way to add modals without wrestling with custom JavaScript. To add a modal to your layout, you first need to include the Hyva modal handle in your layout XML file:
<update handle="hyva_modal"/>

Next, you create a template that handles the logic for showing and hiding the modal. Here's a basic example:
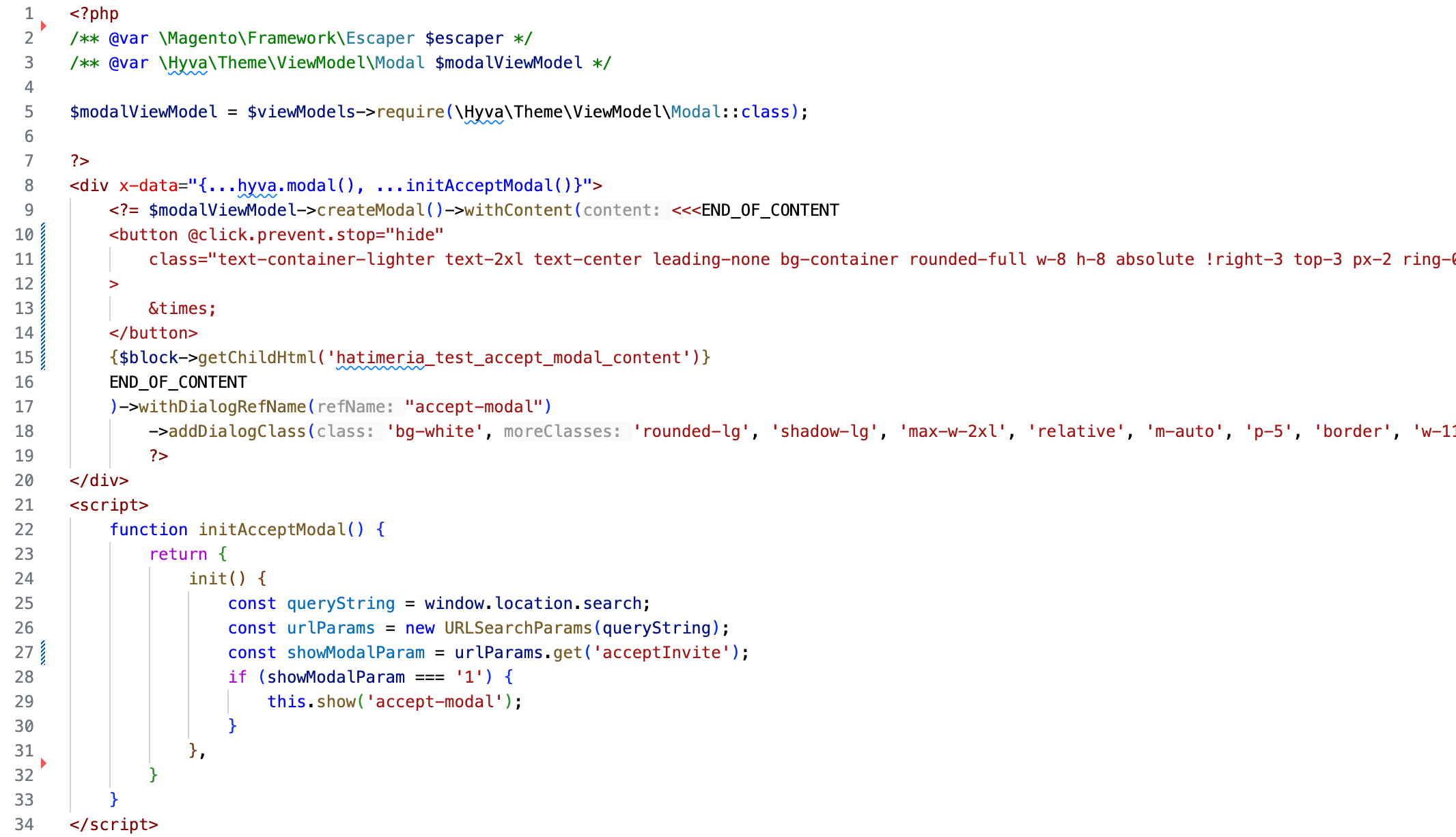
Optionally, you can create a child block in your layout XML to hold more complex content for the modal, which you can then render inside your modal template using:
$block->getChildHtml('your_child_block_name')
Hyva makes it easy to manage modals. The hyva.modal() Alpine.js component provides show() and hide() methods. Also, to open a modal from outside its own Alpine.js context, you can dispatch an event:
window.dispatchEvent( new CustomEvent('hyva-modal-show', { detail: { dialog: 'your-dialog-ref-name' } }) );
Your modal automatically listens for this event. The PHP Modal view model also offers many functions for customizing the modal's behavior and appearance. For more detailed information, it's best to check the official Hyva documentation.
2. Form Validation – No-Hassle Checks
Hyva simplifies client-side form validation. You don't need to write complex JavaScript or extra validation libraries. To create a simple validated form, you can use Alpine.js directives along with Hyva's validation features. Hyva provides a hyva.formValidation Alpine component that you can use. Here's how you can set up a basic form with validation:
- Turn on validation in your layout XML:
<update handle="hyva_form_validation"/>
- Add the
x-data="hyva.formValidation"
directive to your form element.
-
Add validation rules directly in your HTML using attributes like required, type="email", minlength, maxlength, or custom patterns.
-
Override any default message with data-msg-<validator> (e.g. data-msg-maxlength) - %0 will be replaced by the rule’s parameter.
-
Style error states using Tailwind’s state-dependent variants. The invalid: and valid: pseudo-class variants let you highlight inputs based on their validity - no extra JavaScript needed.
-
Display error messages using
field field-reserved
class on the wrapper element.
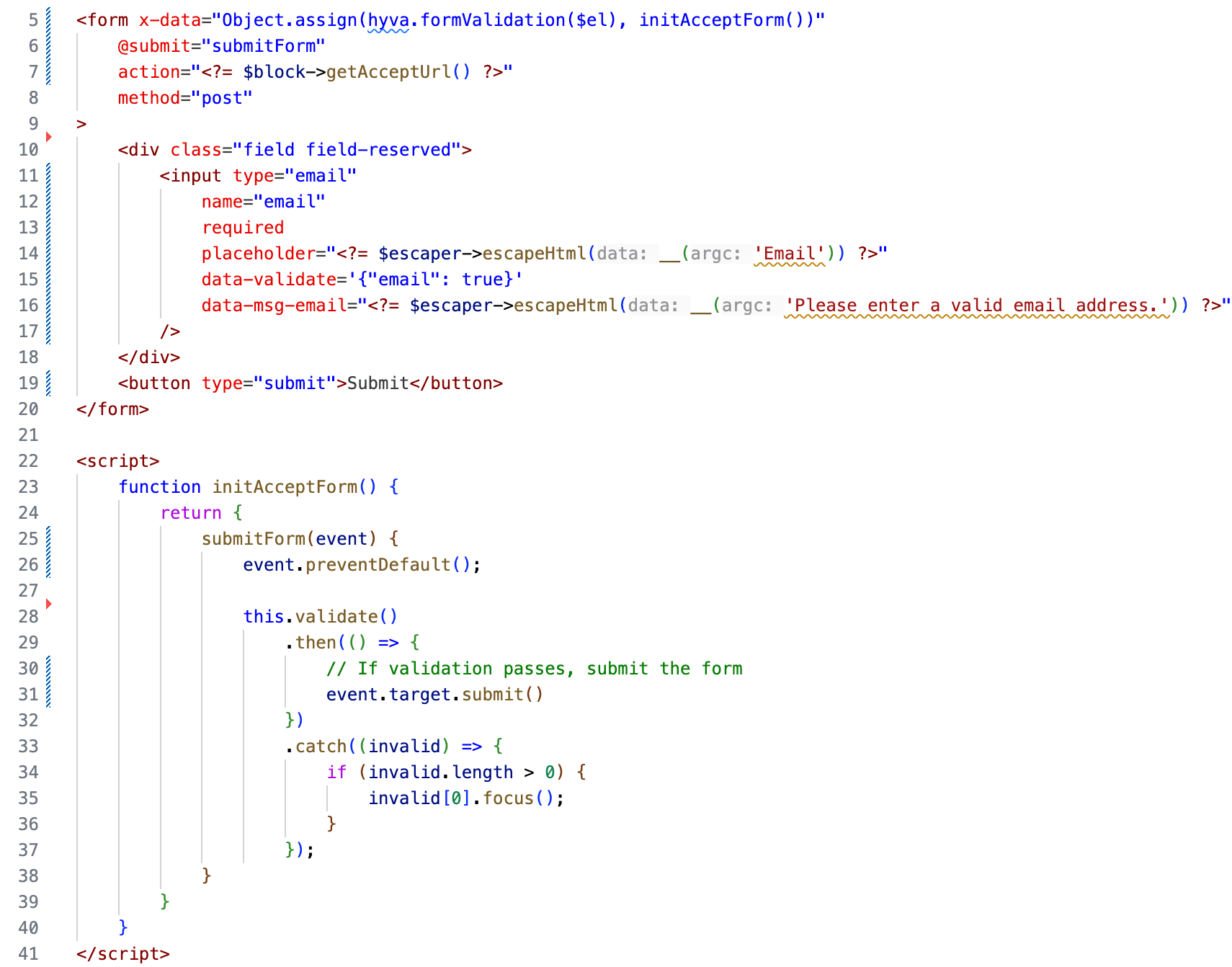
3. Building URLs in JavaScript
Hyvä gives you two handy global variables - BASE_URL and CURRENT_STORE_CODE - so building links and API endpoints is really simple. Instead of hardcoding URLs or passing them from PHP in complex ways, you can use JavaScript. Just plug in these variables wherever you need them, whether you’re linking to a page or calling a REST/GraphQL endpoint.
${BASE_URL}example/path/action
${BASE_URL}/rest/${CURRENT_STORE_CODE}/example/path/action
4. Section Data – Scoped and Clean
Section data is Magento’s way of keeping track of user-specific bits like cart contents or customer info. In Hyva, you simply use the Alpine custom component, and you can write a function inside to run when data arrives. Just listen for the private-content-loaded event on the window, then call receive($event.detail.data) in your handler. Whenever Magento pushes new section data, your function fires and updates your component-no extra AJAX polling or custom event wiring needed.
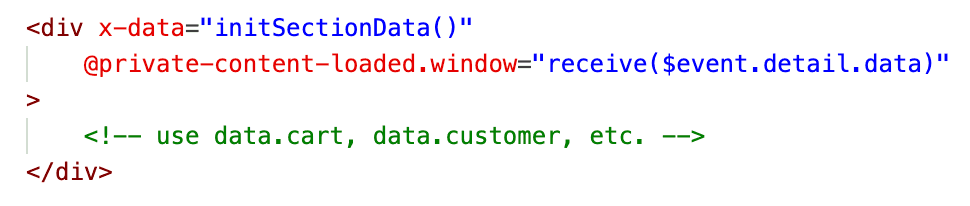
5. window.dispatchMessages() – Notifications
Hyvä includes a handy, Alpine-compatible way to show frontend messages like success, error, or warning notifications. You don't need to add extra libraries for basic toast messages. You can trigger these messages from anywhere in your JavaScript code using the window.dispatchMessages() function.
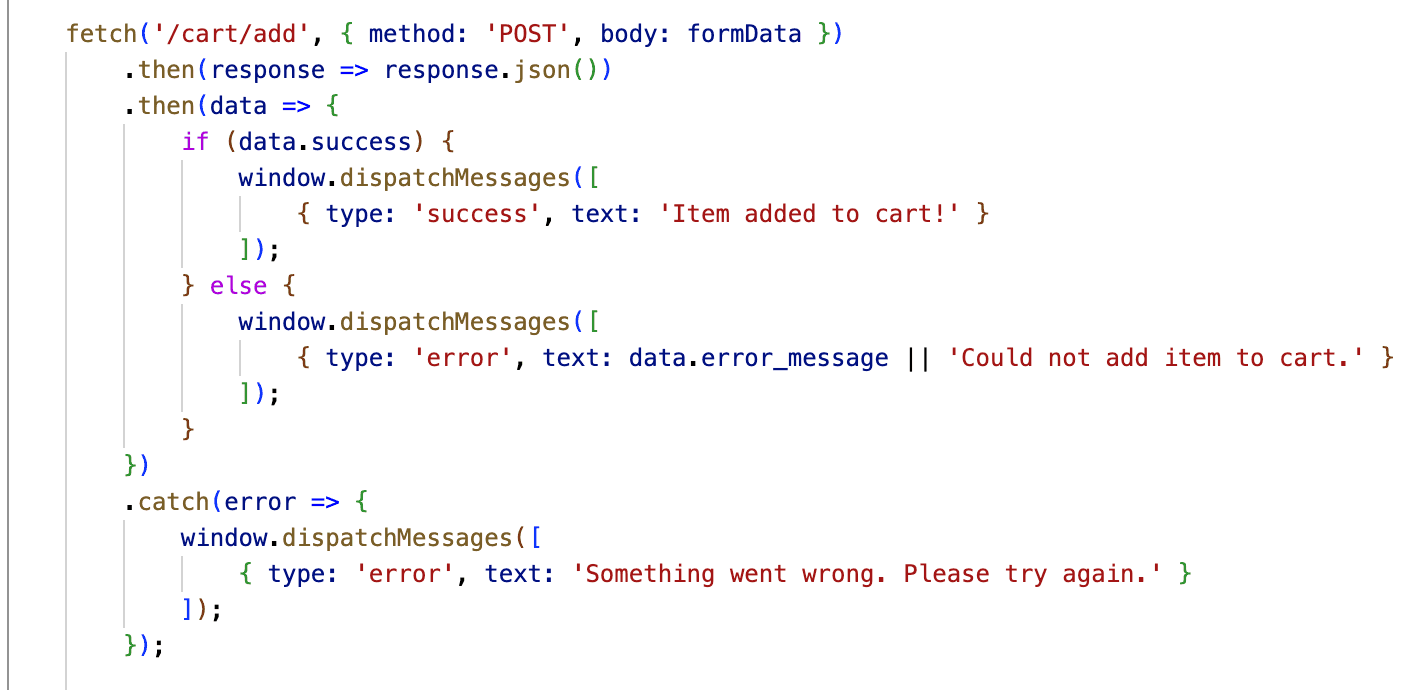
6. GraphQL View Model
Hyvä GraphQL view model makes querying Magento’s GraphQL API feel like magic. Just require the view model in your template, call query(), and Hyva handles the fetch, cache - everything. You can even hook into render events to tweak queries or add fields on the fly.
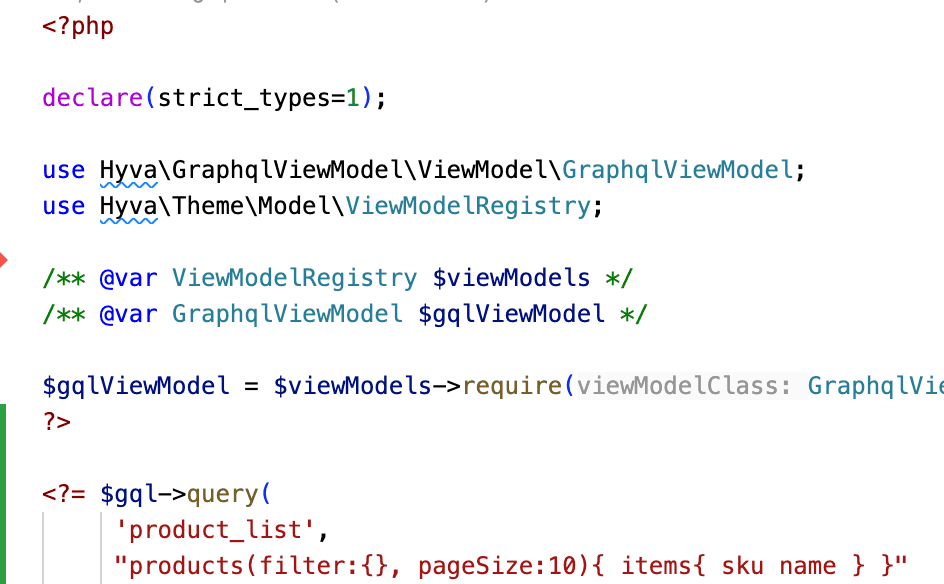
7. Creating a custom SVG Icon View Model
Hyva themes include built-in support for HeroIcons via the
Hyva\Theme\ViewModel\HeroiconsOutline and Hyva\Theme\ViewModel\HeroiconsSolid view models, which is fantastic for quick and consistent UI development.
Example of using default HeroIcons:
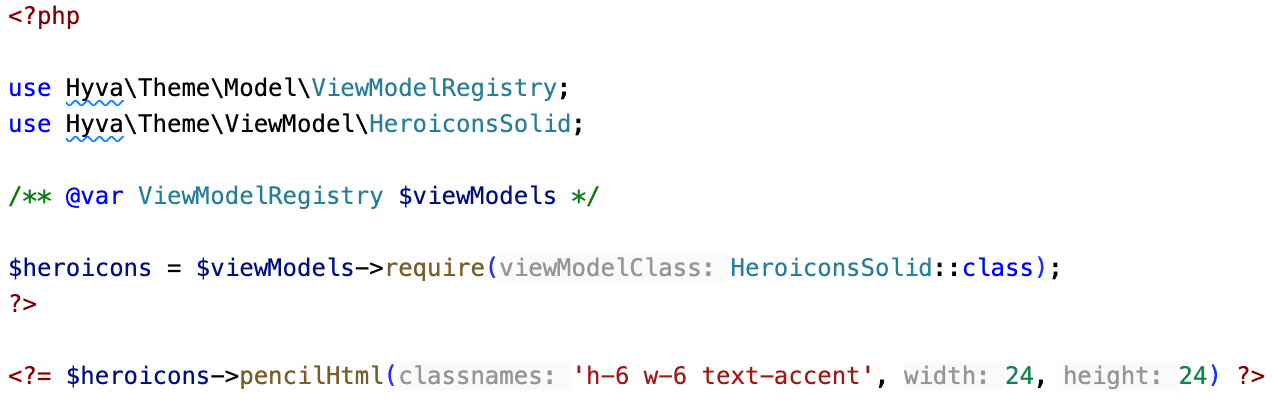
But you often need to use your project's unique SVG icons. Hyva makes it straightforward to integrate your own custom SVG icon sets directly within your theme. This allows you to manage and use your specific icons with the same clean ViewModel approach.
- First, create a folder in your theme at: app/design/frontend/YourVendor/yourtheme/web/svg/
- And upload your custom SVG icon e.g. home.svg
- Next, in your PHTML or template, require the generic view model and call renderHtml() or the magic method matching your filename:
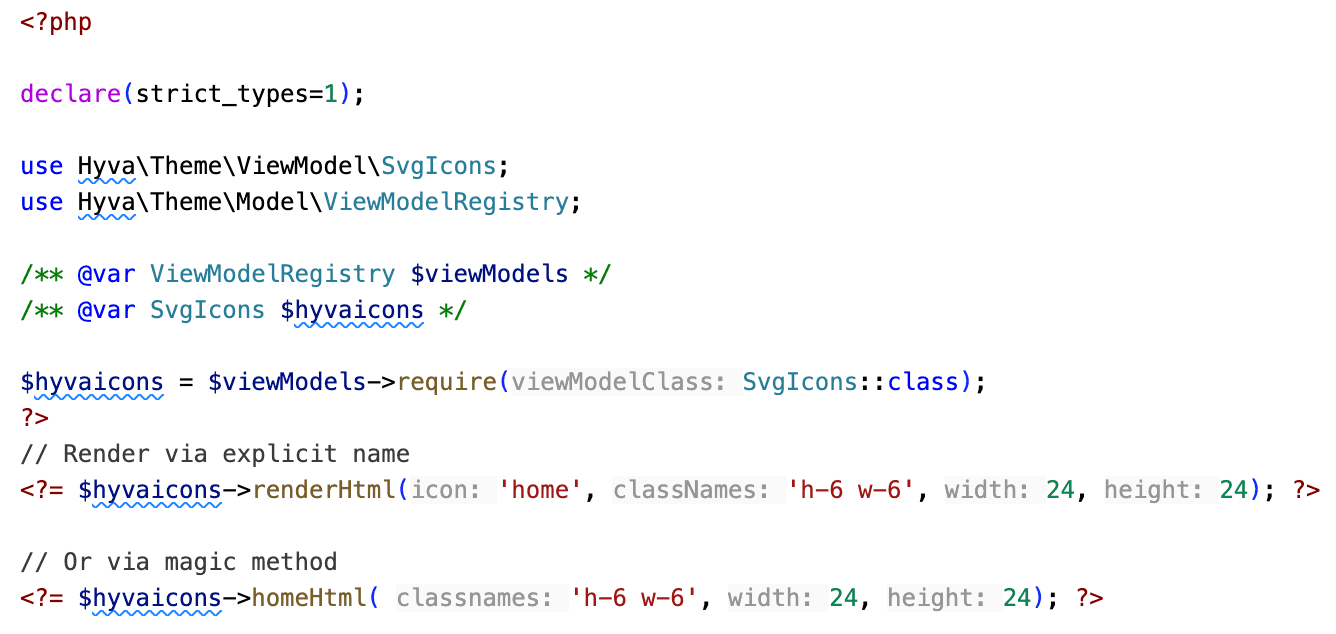
8. Default Hyvä Loading
Show a loading spinner while you wait for data without writing custom markup. Hyvä provides a loading template you can drop into your layout XML and toggle with a simple Alpine flag. Your content only appears once the data is ready, giving users clear feedback that something is happening.
Add this line into your layout XML file:
<block name="loading" template="Hyva_Theme::ui/loading.phtml"/>
And then, toggle “loading” Alpine variable to trigger the loading animation.
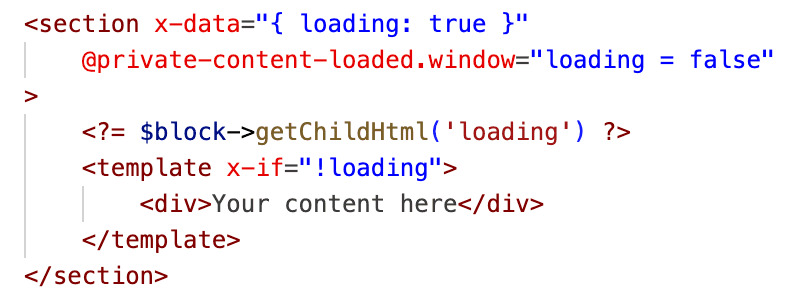
9. Luma Theme Fallback – Mix in Luma When Needed
Sometimes you need the original Luma look—like for the Luma Checkout or special Magento routes. Hyvä offers a fallback module that lets you pick which URLs use the Luma theme instead of Hyvä. First, install it: composer require hyva-themes/magento2-theme-fallback bin/magento setup:upgrade
Then, in the Admin under HYVA THEMES → Fallback theme, enable the feature, set the theme path and list URL parts (e.g., checkout/index). When a user visits those URLs, Tailwind and Alpine won’t load - instead, Magento’s RequireJS and Luma CSS/JS take over.
10. Alpine Store – Share Data Everywhere
Hyva comes with Alpine's global stores enabled right away. If you have data or a state that needs to be shared across many components, like a cart drawer, Alpine’s store system is perfect for you.
For example, in our latest +Shop case study we needed a global manager to handle the state of a custom entity “level”. The "level" needs to be accessible and editable from different parts of the page. Using Alpine Store, we could easily create a global variable to hold the current context and use functions to update it.
In the JS file:
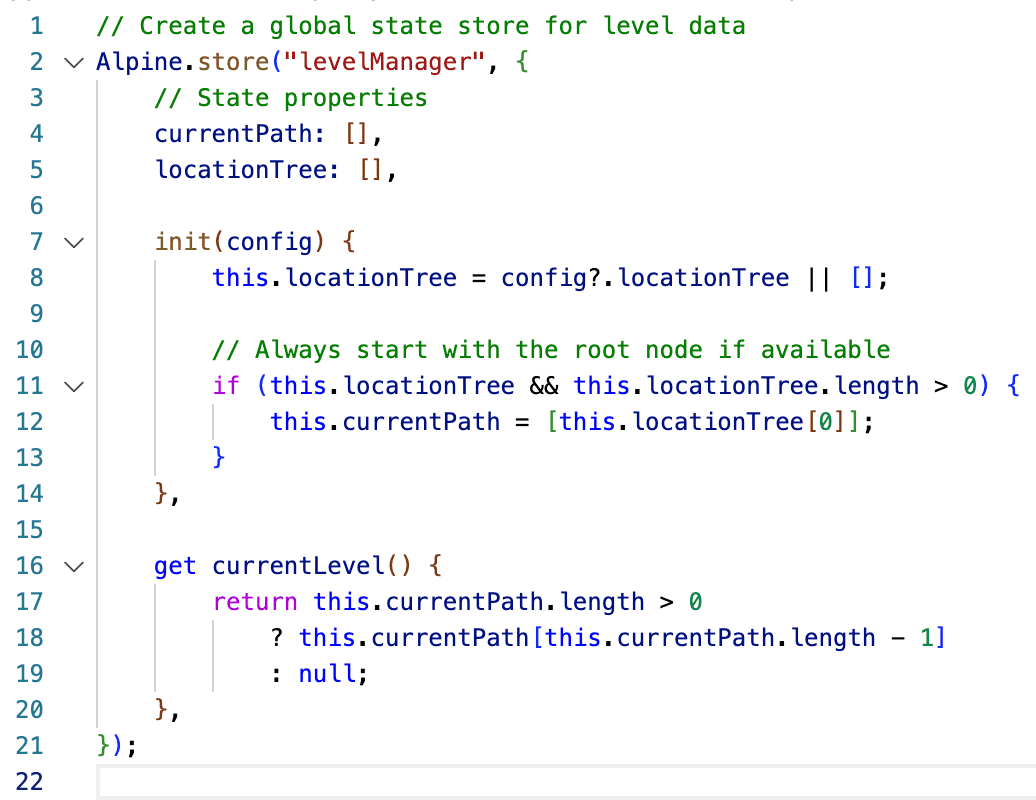
From Real Projects
We used these Alpine.js + Hyva tricks in several Hyvä-based Magento projects, especially in our latest +Shop case study. It helped us:
-
Built many advanced modals in a clean, consistent way using the Hyvä modal helpers.
-
Migrated from Luma to Hyvä by splitting work into two phases: the rest of the site first, then checkout—thanks to the Luma Theme Fallback.
-
Created a lot of custom forms in +Shop; Hyva’s built-in form validation with Alpine made it super simple.
-
Extended section data to include our own custom fields; Alpine integration made reading and updating that data trivial.
-
Needed to show status, progress, and errors on many forms - Hyva’s default toast messages saved us from writing our own notification system.
-
Used Hyva’s GraphQL view model extensively for getting necessary data, with zero hassle.
-
Added loading spinners around dynamic form submissions and data loads - Hyva’s default loading indicator meant no extra markup or JS.
If you're building Magento stores with Hyva, Alpine.js is your best friend. It keeps things simple, fast, and developer-friendly.
Want to see how we used Alpine.js in a full Hyvä project? Drop me a message - happy to share tips or examples.
Would you like to innovate your ecommerce project with Hatimeria?
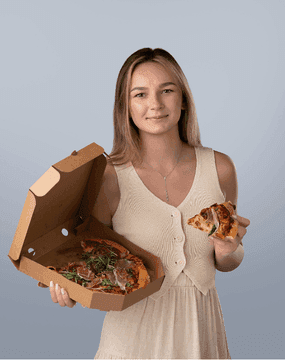
Anita is a passionate Junior Fullstack Developer with 2 years of experience in Flutter, crafting mobile apps and websites. Her skill set also includes expertise in Node.js and Firebase. Now, she's excited to embrace new challenges and grow her career in fullstack development. Privately, Anita loves to make delicious Italian dishes, enjoying experimenting with flavors and recreating authentic recipes that bring a taste of Italy to her home.
Read more Anita's articles