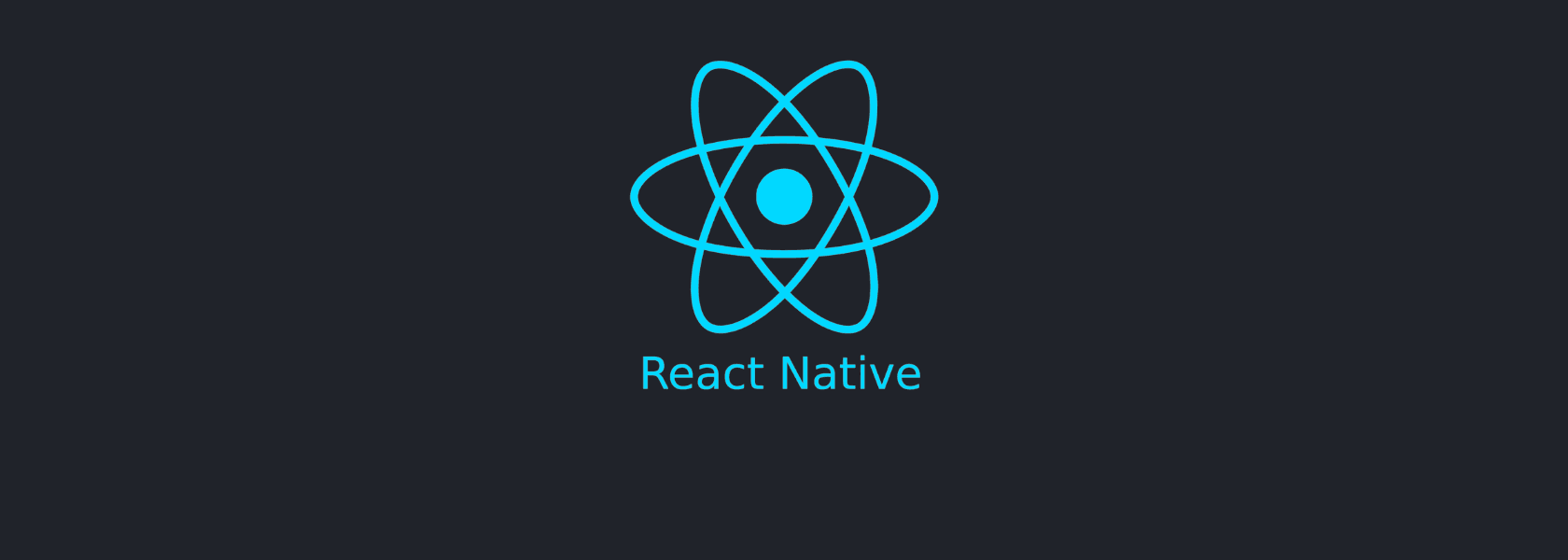
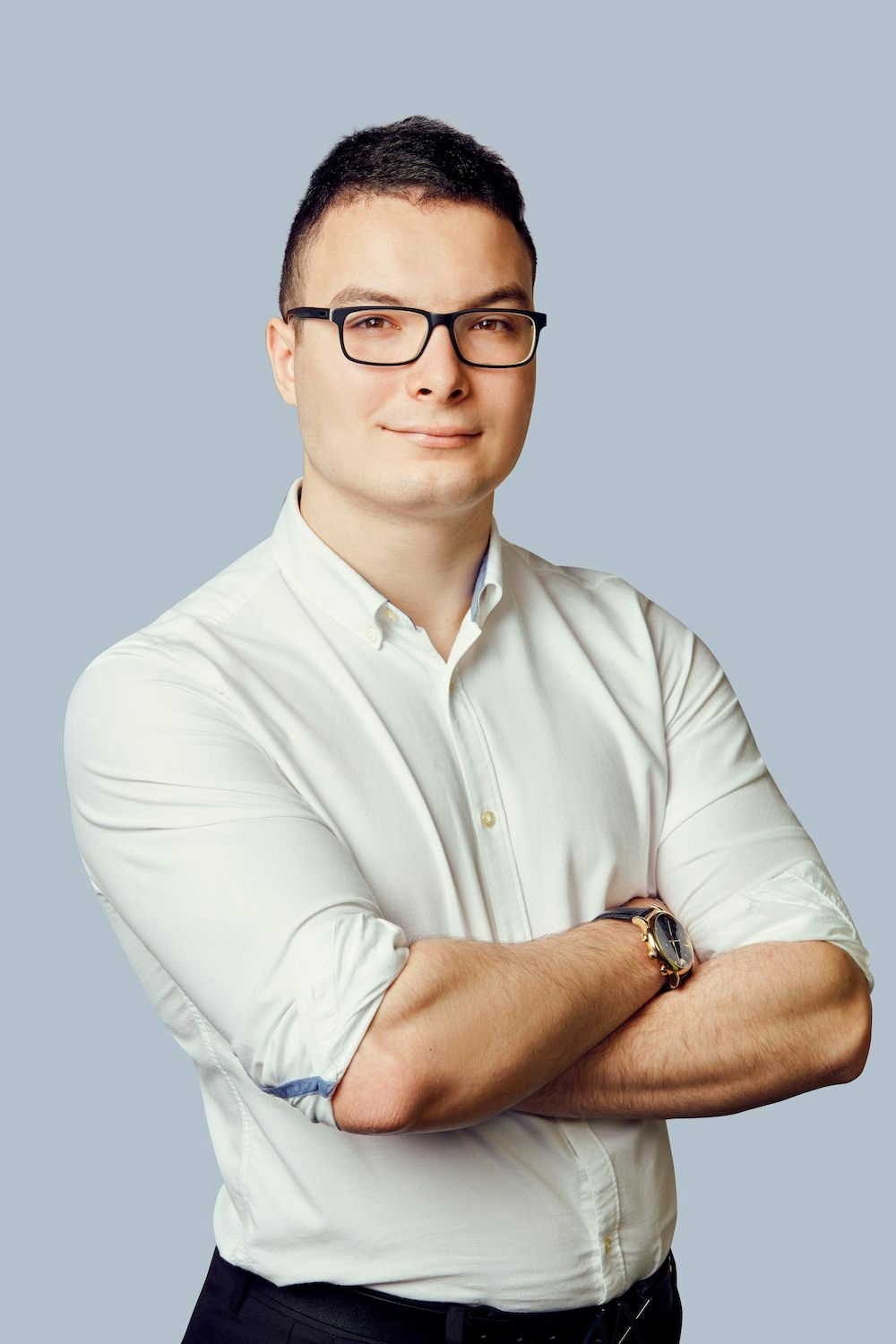
By Dominik
March 02, 2023
Building Better React Native Apps: Lessons Learned from Common Mistakes
React Native is a popular open-source framework that enables developers to create native mobile applications using JavaScript and React. However, like any development process, there are common mistakes that developers make when building React Native applications. In this post we will explore these common mistakes and provide tips on how to avoid them to create more efficient and high-quality React Native applications. Whether you're a beginner or an experienced developer, this post will help you improve your React Native development skills.
The tips included in this post can also be applied when creating web applications using React and other frameworks!
Why Insufficient Knowledge Can Lead to Mistakes
React Native, like any framework, has its own set of rules and best practices that developers need to follow to ensure that their code is efficient, maintainable and bug-free.
One common mistake that developers make when using React Native is a lack of knowledge of its fundamentals. React Native uses a component-based architecture that is similar to React but there are some differences that developers need to understand.
Here are some of the common mistakes that can occur when developers lack knowledge of React Native fundamentals:
-
Not understanding the component life cycle - React Native components have a life cycle that determines when they are created, updated and destroyed. If developers don't understand this life cycle, they may not be able to optimize their applications or handle certain edge cases properly.
-
Not using the correct layout components - React Native provides a set of layout components that are optimized for performance on mobile devices. If developers don't use these components correctly, they may experience performance issues or unexpected behavior.
-
Not understanding the differences between React and React Native - While React and React Native share many similarities, there are some key differences that developers need to be aware of. For example, React Native has its own set of components and APIs that are optimized for mobile development.
Poor Navigation Design
Navigation is a critical part of any mobile application, as it determines how users move through the app and interact with its various features. Poor navigation design can cause issues that can impact the user experience and overall success of the app.
To avoid these common mistakes, it's important to take a thoughtful and deliberate approach to navigation design in React Native. This means using consistent navigation patterns throughout the app, designing a navigation structure that is intuitive and easy to use, handling navigation correctly and optimizing navigation performance.
Here are some common mistakes that developers make when it comes to navigation design:
-
Inconsistent navigation - Inconsistencies in navigation can confuse users and make it difficult to understand how to move through the app. It's important to use consistent navigation patterns throughout the app, such as using a bottom tab bar for the main navigation or a hamburger menu for the secondary navigation.
-
Poorly designed navigation structure - A poorly designed navigation structure can make it difficult for users to find what they're looking for or understand how to use the app. It's important to design a navigation structure that is intuitive and easy to use, with clear labels and hierarchy.
-
Not handling navigation correctly - If navigation is not handled correctly, it can lead to issues such as memory leaks or unexpected behavior. It's important to understand how navigation works in React Native and to use the appropriate navigation library that meets your app's needs. From my own experience I can recommend React Navigation.
-
Not optimizing navigation performance - If navigation is not optimized for performance, it can lead to slow rendering times or other performance issues. It's important to design navigation in a way that is performant and efficient, such as using lazy loading to reduce initial load times.
Maximizing List Usability
In many mobile applications, item lists are a critical part of the user interface, as they allow users to browse and interact with various types of content. However, poor item list design can lead to issues that impact the user experience. In case a list is not optimized, it can lead to slow rendering times or other performance issues.
When it comes to displaying item lists in React Native, there are several components to choose from, including ScrollView, FlatList, and SectionList.
-
ScrollView is the most basic item list component and is used to display a list of items that can be scrolled vertically. However, ScrollView should be used sparingly as it can cause performance issues with large lists. When using ScrollView, all of the items are rendered at once, which can lead to slow rendering times and a sluggish user experience.
-
FlatList is a more performant alternative to ScrollView, designed specifically for long, scrollable lists. Unlike ScrollView, FlatList only renders the items that are currently visible on the screen, which reduces the amount of data being rendered and improves performance. FlatList also includes built-in features for handling data pagination and handling scroll events, making it a popular choice for item list views in React Native.
-
SectionList is similar to FlatList but it adds support for section headers and footers, making it ideal for displaying nested lists with varying item types. Like FlatList, SectionList only renders the items that are currently visible on the screen, which makes it a highly performant choice for displaying large and complex item lists.
When choosing between ScrollView, FlatList, and SectionList for an item list view, it's important to consider the specific needs of the app and the performance requirements. For small lists, ScrollView may be a suitable choice, but for large and complex lists, FlatList or SectionList should be used to ensure optimal performance and user experience.
App Update Strategies
Another common mistake that developers make when building apps with React Native is not considering how updates and new features will be implemented in the app. Without proper planning and preparation, app updates can cause issues for users and create a poor user experience.
One way to address this issue is by using feature flags. Feature flags are a technique that allows developers to toggle features on and off within an app without requiring a new release or update. This approach enables developers to test and roll out new features gradually and in a controlled manner, reducing the risk of introducing bugs or other issues.
To implement feature flags in a React Native app, developers can use third-party libraries or create their own solution using a combination of state management and server-side configuration. With feature flags in place, developers can control which features are visible to users based on various conditions such as user roles, device types or geographic location.
Another important consideration when planning app updates is ensuring that the update process is smooth and seamless for users. This means providing clear information about the update, including the reason for the update, the new features or changes and any necessary steps users need to take. It is also important to test the update thoroughly before the release to identify and fix any issues that may arise.
Don't Ignore Platform-Specific UIs
One of the key benefits of using React Native to build cross-platform mobile apps is the ability to share code across different platforms. However, it's important to remember that each platform has its unique design guidelines and UI components. Failing to use platform-specific UI components is a common mistake in React Native app development that can negatively impact the user experience.
Android and iOS have different design principles and UI components and users are accustomed to the native look and feel of their respective platforms. If an app doesn't use platform-specific UI components, it can feel foreign and clunky, which can lead to a poor user experience and negative reviews.
To avoid this mistake, it's important to leverage the platform-specific UI components that are provided by React Native. For iOS, this might include using the native iOS navigation bar, tab bar and toolbar components, while for Android developers can use the native Android Toolbar, DrawerLayout and BottomNavigationView components. Using these components ensures that the app looks and feels familiar to users on each platform, which improves the user experience.
React Native also provides a number of third-party libraries that can help developers implement platform-specific UI components more easily. These libraries can help ensure that the app's UI follows the design guidelines of each platform and provide a more native experience to users.
Designing for Different Screen Sizes
When building React Native apps, it's crucial to ensure that the app looks and performs well on devices with different screen sizes. Although they are mobile devices, the sizes and resolutions of screens can differ significantly. For example, an app designed for a small screen may appear cramped and difficult to use on a larger screen, while an app designed for a larger screen may appear sparse and empty on a smaller screen.
To avoid this mistake, developers should ensure that their React Native apps are optimized for different screen sizes. One way to achieve this is by using the flexbox layout system provided by React Native. The flexbox system allows developers to create responsive layouts that adjust to different screen sizes. Additionally, React Native provides a number of built-in components such as the ScrollView and FlatList components, which are described above.
Don't Ignore performance issues
Thanks to updates released by its creators, React Native app performance has improved significantly in recent years. However, ignoring performance issues is a common mistake made by developers when building native apps. While it may seem like a minor issue at first, ignoring performance can lead to a frustrating user experience, resulting in negative reviews and fewer downloads.
To avoid these issues, developers should possess an understanding of the component life cycle, code optimization, avoiding unnecessary re-renders, minimizing the use of expensive operations and utilizing asynchronous operations to enhance performance. Although these issues are not specific to React Native, they are rooted in the principles of using React. Given the complexity of this topic, I plan to explore it in greater depth in a future blog post.
Additionally, developers should take advantage of React Native's built-in performance tools such as the Profiler and the DevTools. These tools allow developers to identify and diagnose performance issues in their apps.
Conclusion
In conclusion, React Native is a powerful framework for building mobile applications but it's important to be aware of the common mistakes that developers can make.
We've covered some of the most common mistakes. It's important to keep in mind that these are just a few examples and there are many other mistakes that developers might make.
By avoiding these common mistakes and staying informed, you can create amazing React Native applications that will delight your users and stand the test of time.
Would you like to innovate your ecommerce project with Hatimeria?
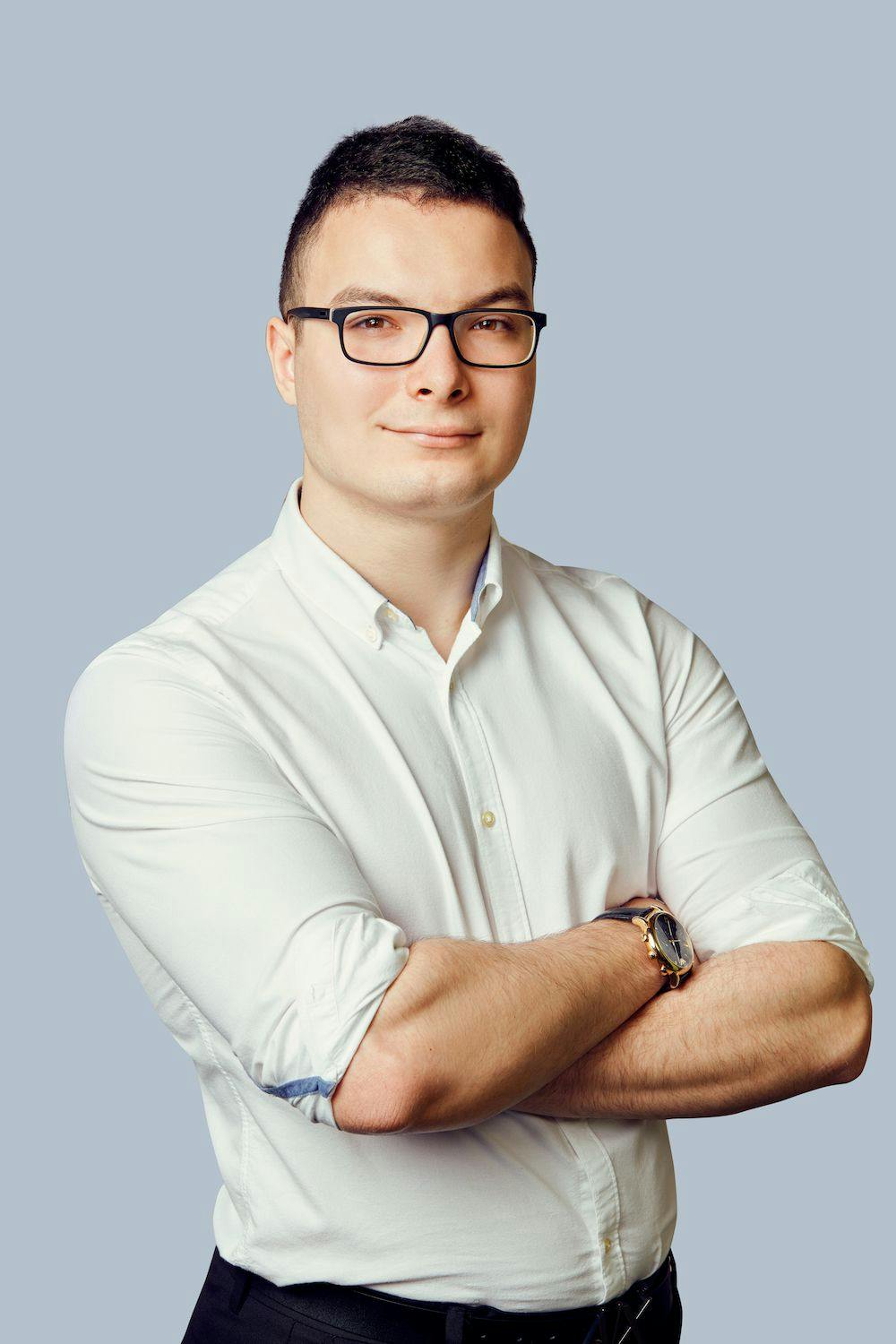
He specializes in technologies such as Node, serverless and web/mobile applications. He is dedicated to expanding his skills and gaining hands-on work experience in the field. His biggest flaws are talkativeness, addiction to coffee and delicious food.
Read more Dominik's articles